注意
本文最后更新于 2024-08-27,文中内容可能已过时。
介绍
公共变量
Vite 在一个特殊的 import.meta.env 对象上暴露环境变量,这些变量在构建时会被静态地替换掉。
加载的环境变量也会通过 import.meta.env 以字符串形式暴露给客户端源码。
为了防止意外地将一些环境变量泄漏到客户端,只有以 VITE_ 为前缀的变量才会暴露给经过 vite 处理的代码。例如下面这些环境变量:
1
2
|
VITE_SOME_KEY=123
DB_PASSWORD=foobar
|
只有 VITE_SOME_KEY 会被暴露为 import.meta.env.VITE_SOME_KEY 提供给客户端源码,而 DB_PASSWORD 则不会。
1
2
|
console.log(import.meta.env.VITE_SOME_KEY) // "123"
console.log(import.meta.env.DB_PASSWORD) // undefined
|
默认情况下,Vite 在 vite/client.d.ts 中为 import.meta.env 提供了类型定义。随着在 .env[mode] 文件中自定义了越来越多的环境变量,你可能想要在代码中获取这些以 VITE_ 为前缀的用户自定义环境变量的 TypeScript 智能提示。
见下面额外配置
.env文件
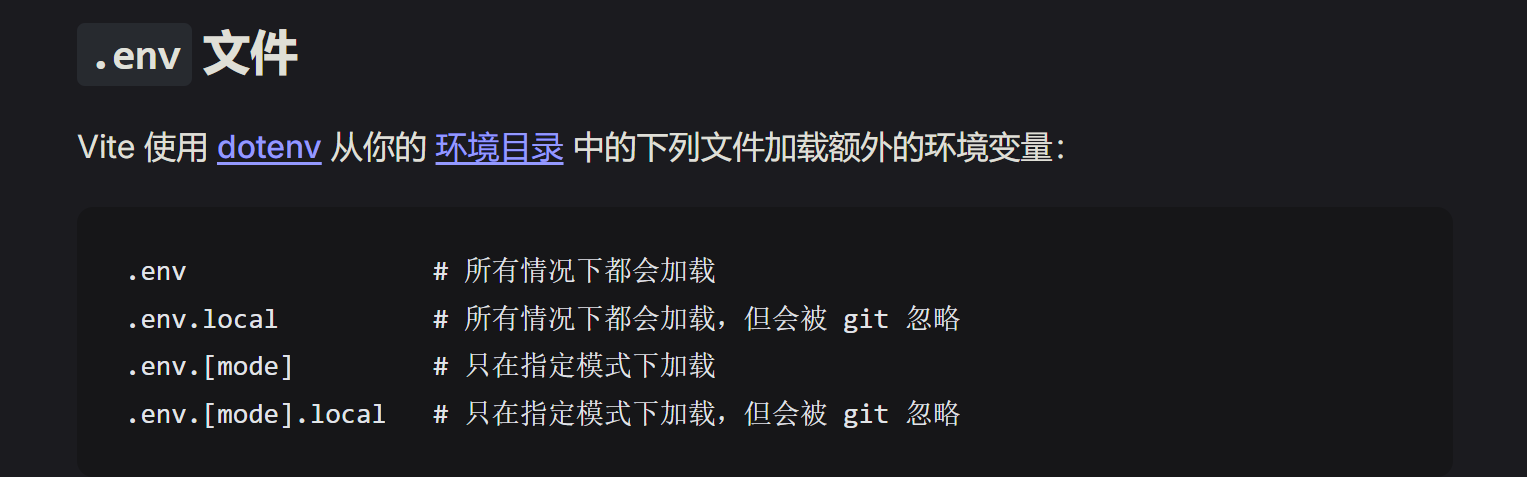
使用
带VITE的普通使用方法
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
|
login.vue
<script setup lang="ts">
import {reactive} from "vue";
const form = reactive({
username: "",
password: "",
})
console.log(import.meta.env)
</script>
<template>
<div class="im_login">
<div class="banner">
</div>
<div class="login_form">
<el-form :model="form">
<el-form-item>
<el-input v-model="form.userName" placeholder="用户名">
<template #prefix>
<i class="iconfont icon-yonghuming"></i>
</template>
</el-input>
</el-form-item>
<el-form-item class="item_password">
<el-input v-model="form.password" placeholder="密码">
<template #prefix>
<i class="iconfont icon-mima"></i>
</template>
</el-input>
</el-form-item>
<el-form-item class="item_action">
<el-checkbox>记住密码</el-checkbox>
</el-form-item>
<el-form-item class="item_btn">
<el-button style="width: 100%" type="primary">登录</el-button>
</el-form-item>
</el-form>
<div class="other_login">
<div class="label">第三方登录</div>
<div class="icons">
<i class="iconfont icon-QQ"></i>
</div>
</div>
</div>
</div>
</template>
<style lang="scss" scoped>
.im_login {
width: 500px;
height: 406px;
background-color: white;
border-radius: 5px;
box-shadow: 0 0 5px 3px rgba(0, 0, 0, 0.08);
overflow: hidden;
}
.banner {
height: 140px;
width: 100%;
background-image: url("https://blog.meowrain.cn/api/i/2024/08/23/LVgCv61724406672895269922.webp");
background-size: cover;
background-repeat: no-repeat;
background-position: center;
}
.login_form {
padding: 20px 80px;
.item_password, .item_action ,.item_btn{
margin-bottom: 6px;
}
.other_login {
display: flex;
flex-direction: column;
align-items: center;
.label {
font-size: 14px;
color: #555;
display: flex;
align-items: center;
width: 100%;
justify-content: space-between;
&::before, &::after {
width: 35%;
height: 1px;
background-color: #e3e3e3;
content: "";
display: inline-flex;
}
}
.icons{
margin-top: 5px;
i{
font-size: 36px;
cursor: pointer;
}
}
}
}
</style>
|
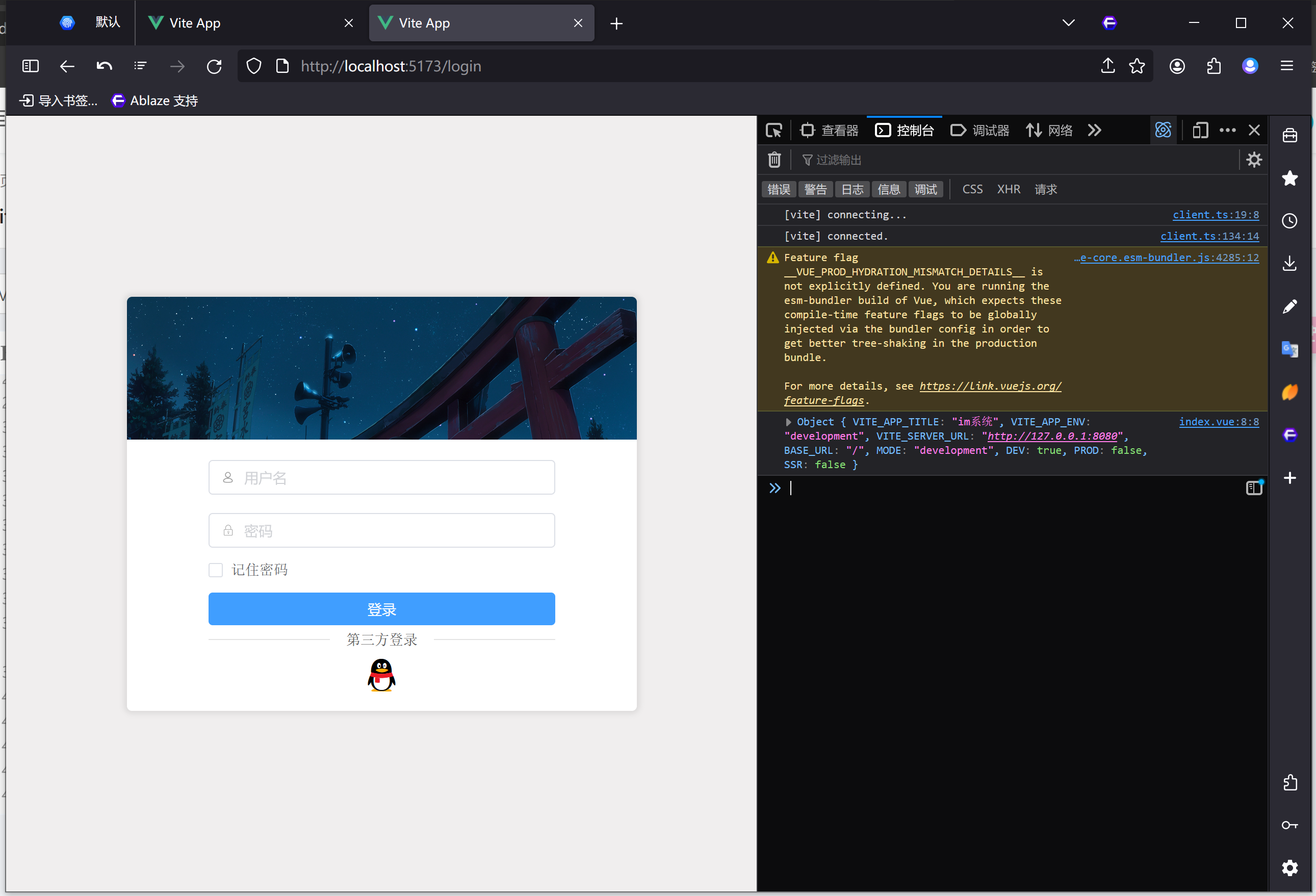
不带VITE的额外配置
在 vite 中对于不支持 import.meta.env来获取变量,我们可以使用 Vite 导出的 loadEnv 函数来加载指定的 .env 文件
比如我们写一个.env文件
1
2
3
4
5
6
7
|
# 页面标题
VITE_APP_TITLE = 管理系统
# 开发环境配置
VITE_APP_ENV = 'development'
VITE_SERVER_URL=http://127.0.0.1:8080
|
然后我们需要在env.d.ts
中这样写
1
2
3
4
5
6
7
8
9
|
/// <reference types="vite/client" />
export interface ImportMetaEnv {
VITE_SERVER_URL: string
VITE_APP_TITLE: string
VITE_APP_ENV: string
}
interface ImportMeta {
readonly env: ImportMetaEnv;
}
|
主要是编写ImportMetaEnv的interface
然后我们在vite.config.ts
中修改defineConfig
函数
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
import { fileURLToPath, URL } from 'node:url'
import {defineConfig, loadEnv} from 'vite'
import vue from '@vitejs/plugin-vue'
import vueJsx from '@vitejs/plugin-vue-jsx'
import type {ImportMetaEnv} from "./env";
// https://vitejs.dev/config/
export default defineConfig(({command,mode})=>{
console.log(mode)
let env:Record<keyof ImportMetaEnv,string> = loadEnv(mode,process.cwd())
console.log(env)
return {
plugins: [vue(), vueJsx()],
resolve: {
alias: {
'@': fileURLToPath(new URL('./src', import.meta.url))
}
},
envDir: "./"
}
})
|
主要是使用loadEnv函数
1
|
function loadEnv(mode: string, envDir: string, prefixes?: string | string[] | undefined): Record<string, string>
|
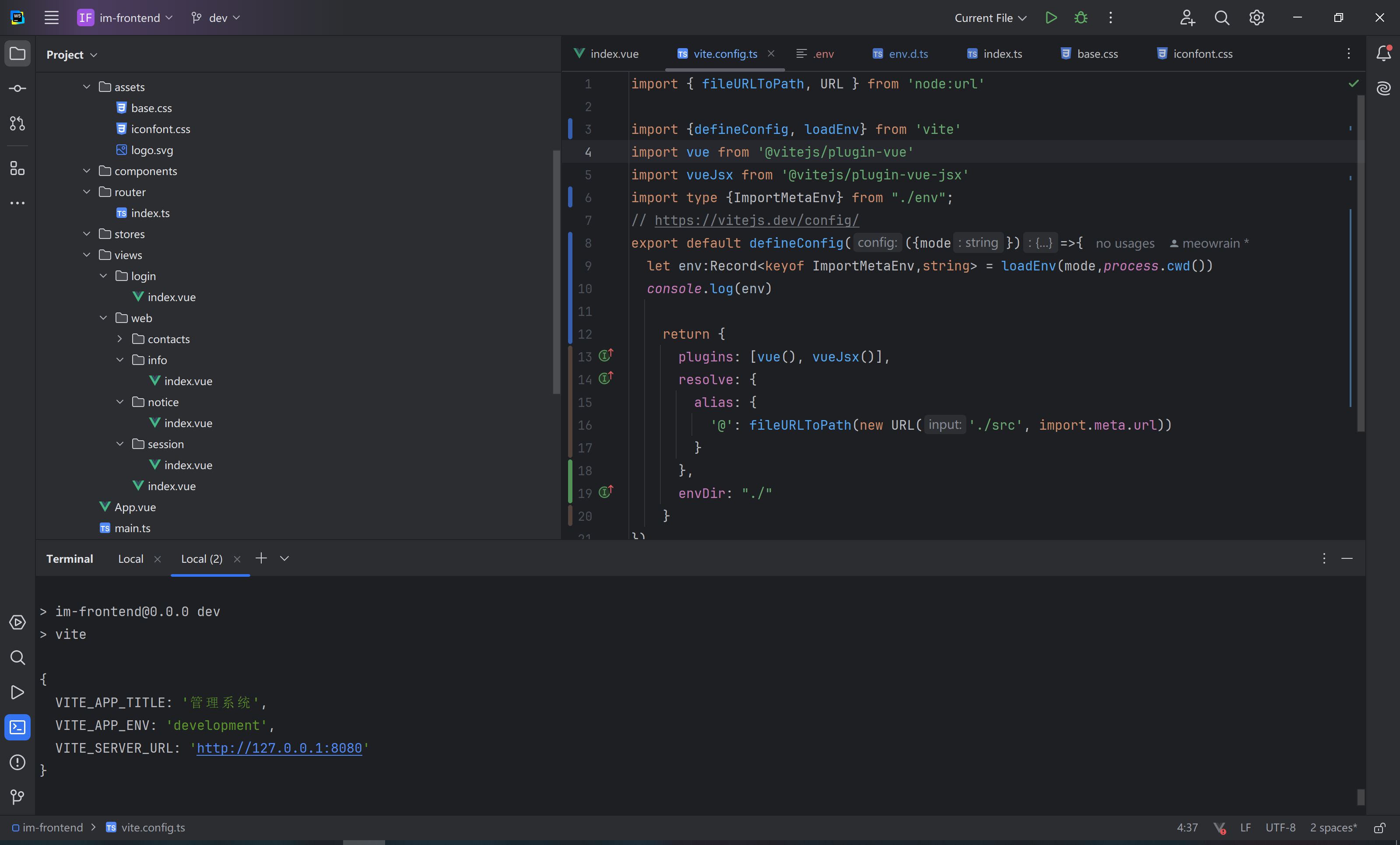