警告
本文最后更新于 2024-05-15,文中内容可能已过时。
使用详情见
https://pkg.go.dev/flag
https://www.liwenzhou.com/posts/Go/flag/
例子
写一个模拟git命令的
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
|
package main
import (
"flag"
"fmt"
"os"
)
func main() {
// 定义子命令
initCmd := flag.NewFlagSet("init", flag.ExitOnError)
commitCmd := flag.NewFlagSet("commit", flag.ExitOnError)
// 为 commit 子命令定义参数
commitMessage := commitCmd.String("m", "", "Commit message")
// 检查是否传递了子命令
if len(os.Args) < 2 {
fmt.Println("expected 'init' or 'commit' subcommands")
printHelp()
os.Exit(1)
}
// 解析子命令和参数
switch os.Args[1] {
case "init":
handleInit(initCmd, os.Args[2:])
case "commit":
handleCommit(commitCmd, os.Args[2:], commitMessage)
default:
fmt.Println("expected 'init' or 'commit' subcommands")
printHelp()
os.Exit(1)
}
}
// 处理 init 子命令
func handleInit(initCmd *flag.FlagSet, args []string) {
initCmd.Parse(args)
if len(args) > 0 && (args[0] == "-h" || args[0] == "--help") {
initCmd.Usage()
return
}
fmt.Println("Repository initialized")
}
// 处理 commit 子命令
func handleCommit(commitCmd *flag.FlagSet, args []string, commitMessage *string) {
commitCmd.Parse(args)
if len(args) > 0 && (args[0] == "-h" || args[0] == "--help") {
commitCmd.Usage()
return
}
if *commitMessage == "" {
fmt.Println("commit message is required")
commitCmd.Usage()
os.Exit(1)
}
fmt.Printf("Commit with message: %s\n", *commitMessage)
}
// 打印帮助信息
func printHelp() {
fmt.Println("Usage:")
fmt.Println(" git init Initialize a new repository")
fmt.Println(" git commit -m Commit changes with a message")
}
|
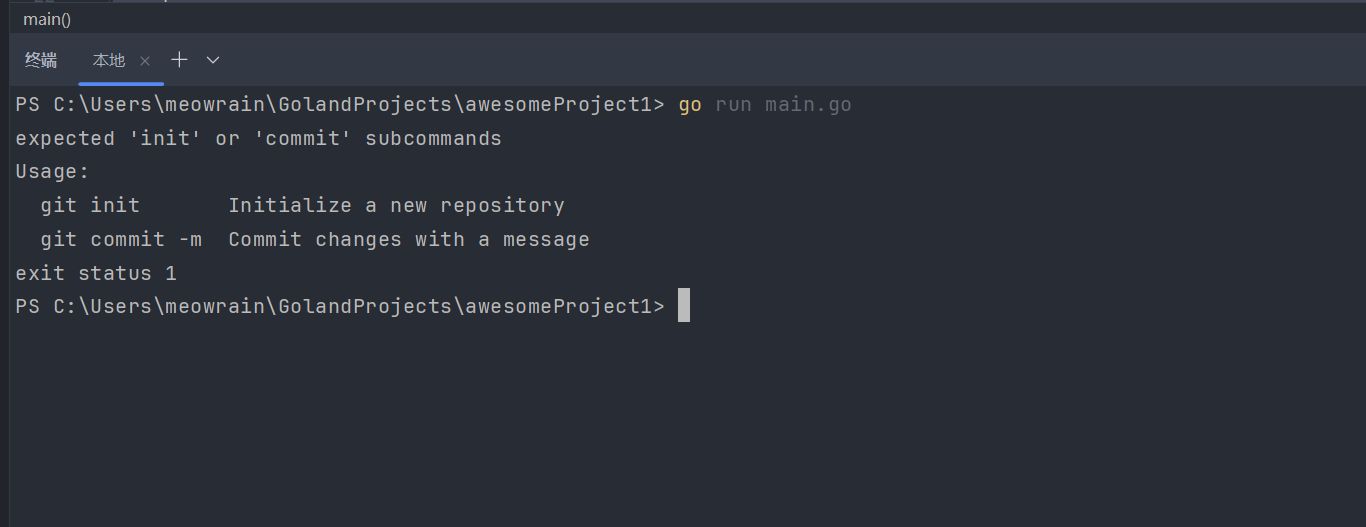
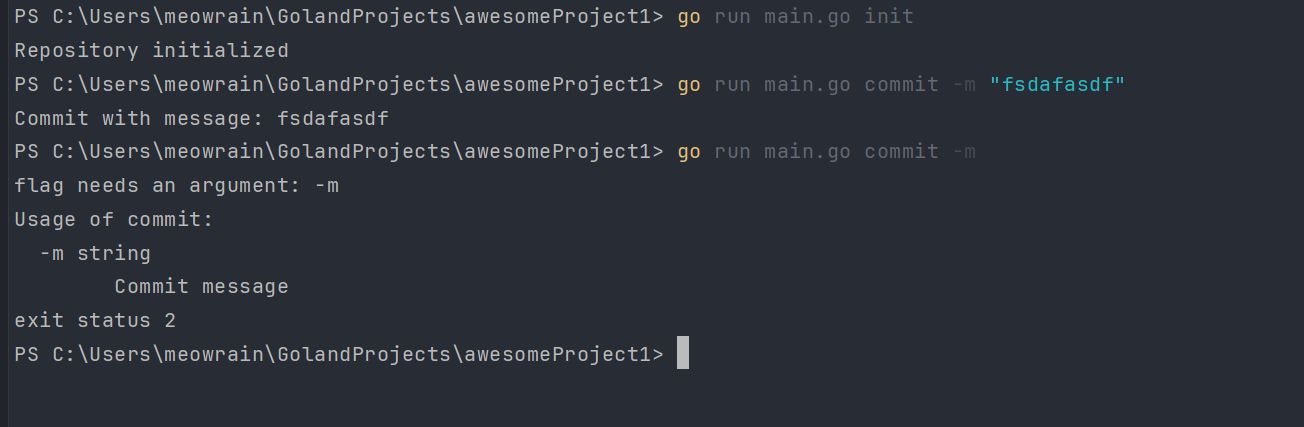